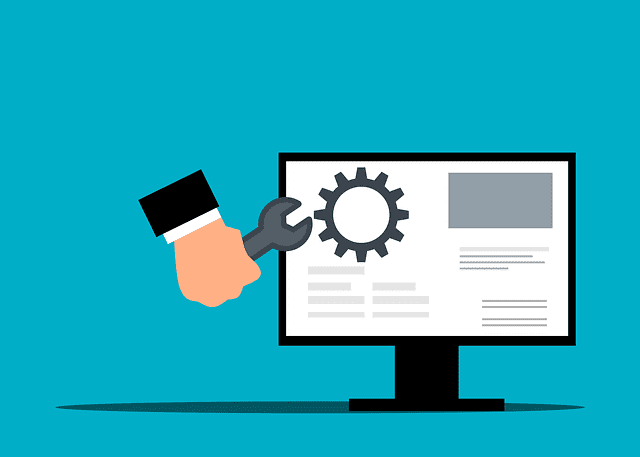
Modern Software Development Life Cycle Part 1
I have been doing JavaScript development for many years now but it was always included as part of some other server side code that was easy to manage. When I started working on Office365 and doing 100% client side code I have struggled with the best way to manage the artifacts that are being created. With server-side code we just used Visual Studio and that would handle our connection to version control and a plethora of other tools to help us write better code. When things changed to working in Office365 I started out by adding the artifacts to a library in SharePoint and then editing them with SharePoint Designer. I know, I know, SharePoint Designer is not a good code editor but in regards to save performance, SharePoint Designer behaves better than anything else out there. The problem with this is that the code that I am creating is not stored in a centralized source repository. Also, if I wanted to get the code into the repository it was a manual effort to copy the files back to my machine and then check everything in. Dealing with multiple developers working on a project could be rather tricky since we were working on the exact same files. There have been times were changes have been temporarily lost due to a save that did not include the changes from other developers. Because of this I always make sure the library I am using has versioning turned on and sometimes I’ll require checkout depending on the likelihood of multiple devs working on the same files. Another problem with this approach is that there is no way to run any code improvement processes. If I want to use TypeScript for example, your code has to be on a local drive for the TypeScript compiler to do its thing. If you have try to run it on a mapped drive through WebDav, TypeScript complains. There are also code tests, bundling, and minification that are tricky to run directly from a SharePoint library. With the introduction of the SharePoint Framework I have realized that I needed to figure out how to best handle the client-side pieces in regards to the Software Development Life cycle. This series of blog posts will cover those topics.
-
Part 1 - New Tools and Setup – This post
-
Part 2 - Continuous Integration
-
Part 3 - Integrating with the SharePoint Framework
New Tools Required
With the “modern” development methods, yes this will be obsolete before too long, a new way of looking at the code was required. What I have found is my experience with what works for me. I am open to suggestions that you may have found helpful that I could integrate as this is a work in progress. In this blog post I will not be going over the SharePoint Framework in any depth. The approach we are talking about today uses some of the similar tools and concepts and I will have a follow-on article on how to integrate both client-side code and the SharePoint Framework into some cool areas for our SDLC including continuous integration and automated deployments. For today though we are going to talk about how we can simplify our processes. We will be using Visual Studio Code as our editor but this is not required. You can use whatever editor you so choose. For the nice integrations that are included is why I chose this editor. We are going to be using Visual Studio Team Services as our version control repository and we will be using gulp as our task processor. Another big factor in determining our toolset is that we need something that is not dependent on a particular operating system. So, the examples that we will be showing will work on a Windows machine, OSX, and Linux.
Visual Studio Code
There are several reasons why we are using Visual Studio Code for our process but the big ones boil down to the following:
-
It is Free
-
Not OS Specific
-
Nice integration with Git
Sure, other tools can offer this as well, but these are the big reasons I have landed with this tool. Visual Studio Code is nice because, as part of the interface, we have easy integration with our version control system and some other nice auto complete features.
Visual Studio Team Services
We are using Visual Studio Team Services because it adds a lot of cool features and is super cheap to be able to use. One nice part that we will be taking advantage of is the fact that when setting up a project we can designate that VSTS will use Git as the backend for the repository. Why do we care? Because Git allows us to meet one of the goals in that we can easily integrate access from any OS plus connecting with Git for automation is a lot easier than using the standard TFS setup. As mentioned we will talk in another post on how we can use the continuous integration and deployment features of VSTS, this is some cool stuff.
Gulp
Gulp is a JavaScript based build system. Now there are several other JavaScript based systems that we could use as well. I have found Gulp to be fairly easy to use and work with while also being the chosen system for working with the SharePoint Framework. So, using Gulp means we are using the same software so we can reuse the same skills. Gulp allows us to automate builds as mentioned but in our case, we will be using it manage the deployment of our code.
Let’s Build Something
Now that we have the basics covered let’s get to the fun stuff. What we are going to be doing is we will have a pretty basic application that will show what we can do. For this we need to make sure that you have the following prerequisites installed on your machine.
-
NodeJS – The LTS(Long Term Support) version is most likely what you need and you can install it with the defaults
-
We will run the following command from a Node.js command prompt, start one after NodeJs finishes installing
-
Setup your project folder
-
Once there, run
-
npm init - Fill out the prompts. This will setup your project file package.json
-
npm i gulp gulp-util Fabian-Schmidt/gulp-sharepoint-sync --save-dev – This will add gulp and other needed code to our project.
-
Now open your editor
-
-
In the root of your project folder create a file called gulpfile.js
-
If you are using Git as your repository create a file called “.gitignore” This fill will tell Git to ignore certain items like the node_modules folder
- Add the following text to the file “node_modules” without the quotes
-
With the basics out of the way let’s start adding some code. For this I am going to create a src folder. For our purposes, it doesn’t really matter what type of content we are going to put in it so I am going to add a few html files, a sub folder for images, and a sub folder scripts. The folder structure looks like this,
With our files in place we need to create our gulp file to handle pushing everything to SharePoint. Create a new file called gulpfile.js. To handle syncing our files to SharePoint we will be using some code contributed to the community from a git hub repo. This one handles what I want it to do and does it pretty well. The repo is at https://github.com/Fabian-Schmidt/gulp-sharepoint-sync. There are instructions there as well on how to use this. So we don’t have to store a username and a password inside of our code in version control I will be using the ACS, Access Control Services, to handle the access. I have added a config.json file to hold the client and secret so I don’t have to hard code those in files I’ll be showing you. The instructions on the git hub repo explain how to get a client id and secret. Also I have an article, Using Workflows to Perform Elevated Actions, that also explains how to get this as well.
Here is the content of our gulpfile for reference.
-
var gulp = require('gulp');
-
var gutil = require('gulp-util');
-
var spSync = require('gulp-sharepoint-sync'); //https://github.com/Fabian-Schmidt/gulp-sharepoint-sync
-
var config = require('./config.json');
-
var source = "src";
-
var destination = "/Demo/Gulp";
-
var conn = spSync.create({
-
authenticationMethod: 'ACS',
-
auth_clientId: config.client_id,
-
auth_clientSecret: config.secret,
-
parallel: 5,
-
log: gutil.log,
-
logLevel: 2
-
});
-
gulp.task("deploy-all", function(callback) {
-
var globs = [source + '/**'];
-
return gulp.src(globs, {
-
base: source,
-
buffer: false
-
}).pipe(conn.newerOrDifferentSize(destination)) // only upload newer files
-
.pipe(conn.dest(destination));
-
}) gulp.task("watch", function() {
-
var globs = [source + '/**'];
-
gulp.watch(globs, function(event) {
-
gulp.src(globs, {
-
base: source,
-
buffer: false
-
}).pipe(conn.newerOrDifferentSize(destination)) // only upload newer files
-
.pipe(conn.dest(destination));
-
})
-
});
As you can see our gulpfile is not super complex we are defining two tasks for gulp. The first is called “deploy-all”, this task will sync all the files in the “src” folder and all sub folders due to the “**” with Demo/Gulp folder in the SharePoint site. You can change where the files come from by changing the base value from src on line 5 to whatever folder you use. To change the deployment location, change the value on line 6. This can be an existing folder in a library or if it doesn’t exist the sync will create the necessary structure. The second task, “watch”, will perform the sync as well but it will sync the files as we are changing them instead of forcing us to manually run the task each time. This is the coolest part of the setup. This task gets us the benefits we have discussed. To run this, we need our node.js command prompt. Make sure you are in the root of your project folder and type “gulp watch” This will tell gulp to run the watch task and when we modify a file in the src folder it will pick it up and sync it to SharePoint.